How to Create an Audio Sample Player in React
Ever wanted to build your own audio sample player in React? Whether you're a music producer, sound designer, or just someone who loves working with audio, creating a custom player can give you more control over how you share and experience sounds. In this blog, I'll show you how to create an audio sample player using @wavesurfer/react.
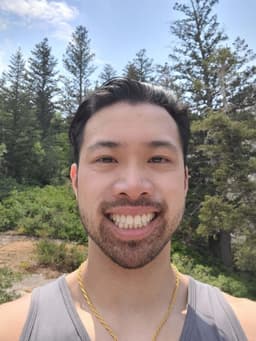
Jordan Wu
3 min read·Posted
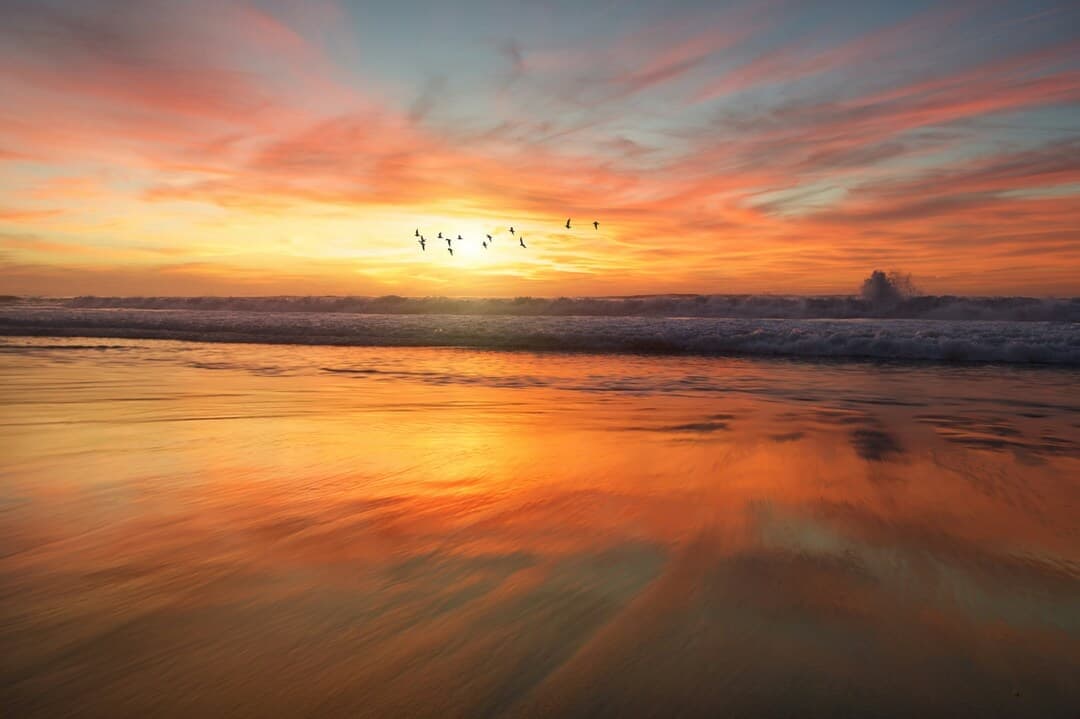
Table of Contents
What is a Audio Sample Player
An audio sample player is a tool used to load, play, and manipulate audio samples, including drums, loops, vocals, synths, one-shots, effects, and instruments. Widely used in music production, sound design, and live performances, it allows users to trigger sounds, adjust pitch and tempo, apply looping, and map samples to keys or pads for seamless playback. These players are essential across various creative industries, enabling musicians, producers, and content creators to explore new sounds and enhance their projects with dynamic audio elements.
Wavesurfer.js
Wavesurfer.js is a lightweight JavaScript library for visualizing and interacting with audio waveforms in web applications using HTML5 and the Web Audio API. It enables customizable waveform displays, audio playback controls, and features like markers, looping, and spectrograms. With support for plugins and responsive design, it’s widely used in music players, podcast tools, and audio editing applications, making it a powerful choice for web-based audio visualization and manipulation.
Create Audio Sample Player React Component
The @wavesurfer/react library makes it easy to integrate Wavesurfer.js into React applications. We’ll use it to build a simple audio sample player component that plays back sound samples. This component will be especially useful for sound designers looking to share their samples with creators.
First install all the dependencies for this component.
pnpm add wavesurfer.js @wavesurfer/react
Next create the audio sample player component.
import { useState } from 'react'
import WavesurferPlayer from '@wavesurfer/react'
import { PauseIcon, PlayIcon } from '@/components/icons'
import WaveSurfer from 'wavesurfer.js'
type AudioSamplePlayerProps = {
url: string
name: string
}
export default function AudioSamplePlayer(props: AudioSamplePlayerProps) {
const { url, name } = props
const [wavesurfer, setWavesurfer] = useState<WaveSurfer | null>(null)
const [isPlaying, setIsPlaying] = useState(false)
const handleReady = (ws: WaveSurfer) => {
setWavesurfer(ws)
setIsPlaying(false)
}
const handlePlayPause = () => {
wavesurfer?.playPause()
}
const handleFinish = () => {
wavesurfer?.seekTo(0)
setIsPlaying(false)
}
return (
<div className="!mt-6 flex flex-col text-center">
<div className="flex justify-center">
<button className="w-[24px]" onClick={handlePlayPause}>
{isPlaying ? <PauseIcon size={20} /> : <PlayIcon size={20} />}
</button>
<WavesurferPlayer
backend="WebAudio"
height={35}
width={250}
cursorWidth={0}
waveColor="#a1a1a1"
progressColor="#fefefa"
url={url}
onReady={handleReady}
onPlay={() => setIsPlaying(true)}
onPause={() => setIsPlaying(false)}
onFinish={handleFinish}
/>
</div>
<p className="!m-2 text-sm">{name}</p>
</div>
)
}
Now to use the component.
<AudioSamplePlayer url="/audio.wav" name="Melodic Loop - 178bpm Bm" />
Medloic Loop - 178bpm Bm
Summary
And that’s it, you’ve successfully built an audio sample player component! This is a powerful way to share sounds on a website and is widely used in applications for music production, sound design, and content creation. Platforms like Splice utilize audio sample players to showcase a vast library of sounds. Allowing users to preview and explore new samples before downloading. Whether you're browsing for new sounds or purchasing sound packs from designers, audio sample players are an essential tool in the creative industry.
Hope you found this blog post helpful and feel inspired to explore new sounds! Here are a few of my favorite random sounds! Enjoy!
BA - Donk Eb
Bass Loop
FX - Atmos Filler
Vocal - Run it
FX - Siren
FX - Glass Break
Synth Chord
BA - 808
BA - Sub
BA - DWILLY
Drum - Snare
Drum Build Loop
Sample Loop
BA - Growl